External pricing page
The inTandem platform offers a user-friendly billing system, enabling partners to efficiently manage client payments. This comprehensive system supports the entire subscription lifecycle, including trial periods, active subscriptions, cancellations, and expiration. Additionally, it facilitates the handling of extra purchases, one-time transactions, and subscription add-ons.
Partners have the flexibility to design their own pricing pages, seamlessly integrating these into the platform's billing process for a more tailored experience."
Flow
- A user clicks a 'Change Plan' button or similar.
- They select a plan on the pricing page.
- The user goes to the checkout page to complete the purchase and enters payment details if it's their first buy.
- The billing system processes the payment.
- After successful payment, the user gets a success message.
- Clicking 'Done' takes the user to a specific page for successful transactions.
- If the payment fails, the user is redirected to a different page for failed transactions.
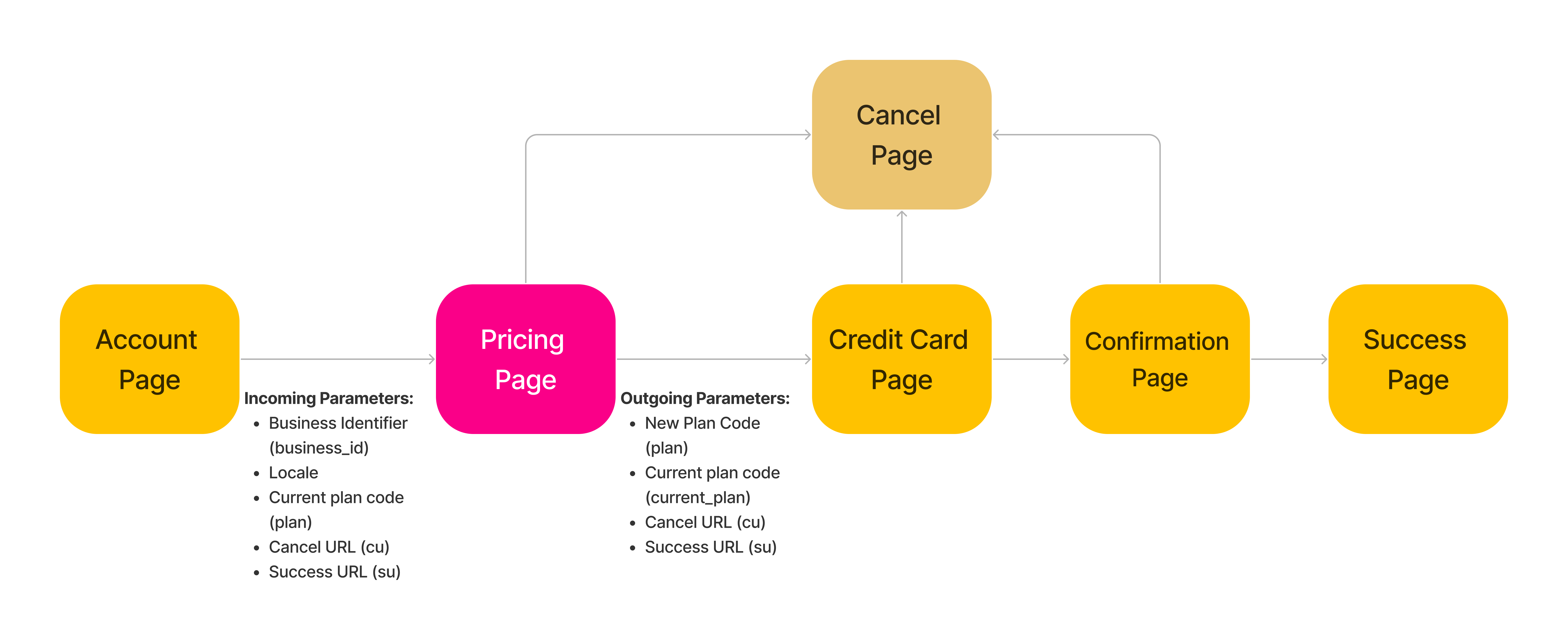
Implementation
Step 1 - Configuration Requirements
To utilize the plug-and-play feature, partners must be configured with the following options:
- You should get a list of available plan codes from vcita.
- Provide the URL for the external pricing page.
Step 2 - Designing an Effective Pricing Page
The pricing page serves as a crucial touchpoint to persuade clients to choose the most suitable plan. It should display various plan options along with their benefits, enabling users to make an informed subscription choice.
Upon a user clicking the purchase call-to-action (CTA), they are redirected to your pricing page. This redirection includes several key parameters (all are query parameters):
- Digest Incoming Parameters:
Upon load, your code should digest the incoming params:
-
Business Identifier (business_id): A unique string identifying the business.
-
Locale: The user's identified locale.
-
Current Plan (plan) : The plan currently used by the business (if apply).
-
Cancel URL (cu): Redirects users who opt to cancel the process and retain their current subscription.
-
Success URL (su): Redirects users upon successful new subscription purchase.
Sample URL : https://yourdomain.com/pricingpage?business_id=123&plan=premium&cu=cancel&su=myaccountpage
- Implement packages
Your page should display the available packages, their features, and corresponding packages. Once selected the page should redirect back to vcita carrying the following outgoing parameters (all are query parameters):
- New Plan Code (plan): The code of the plan selected by the user.
- Current plan code (current_plan): the plan user is replacing (if received)
- Cancel URL (cu): Redirects users who opt to cancel the process and retain their current subscription.
- Success URL (su): Redirects users upon successful new subscription purchase.
-
Implement cancel
Your pricing page should allow users to cancel the purchase, using the provided Cancel URL (composed from your vcita domain and the cu param)Sample URL: https://YOUR_VCITA_DOMAIN/[CANCEL_URL]
-
Implement success
Your page must facilitate the next step of the purchase process by directing the user to a specific, predefined URL. Additionally, it is essential to include both the Cancel URL and the Success URL in this redirection.Sample URL: https://YOUR_VCITA_DOMAIN/paygateproxy?selectedplan=basic¤tplan=premium&cu=cancel&su=success
Additional Notes:
- Authentication: This flow does not require authentication between the product and the pricing page. When users return to the product to complete the purchase, the current session continues. If the session times out, users are redirected to the login page, and post-authentication, they are redirected back to the checkout page.
- Plan Code Consistency: The new plan code must correspond to an existing plan in the product. The purchase flow will fail if this is not the case.
Pricing page example
Full Pricing Page Example for download.
Page HTML:
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Sample Pricing Page</title>
<link rel="stylesheet" href="styles.css"> <!-- You can style the page with a CSS file -->
</head>
<body>
<div class="header">
<a href="https://YOUR_VCITA_DOMAIN/app/null" id="cancel">x</a>
<img src="logo.png" id="logo">
<h1>Sample Pricing Page</h1>
<div class="paragraph-container">
<p>The pricing page serves as a crucial touchpoint to persuade clients to choose the most suitable plan.<br>
It should display various plan options along with their benefits,
enabling users to make an informed subscription choice.</p>
</div>
</div>
<div class="pricing-container">
<div class="pricing-plan" id="business4">
<h2>Essential</h2>
<div class="price" id="essential-price">
<strong>$24/Month</strong><br>
Get 2 months free when billed annually!<br>
($29 /monthly)
</div>
<a href="https://YOUR_VCITA_DOMAIN/app/null?plan=business4&billing=monthly&current_plan=null">
<button class="upgrade-button">Get it now</button>
</a>
</div>
<div class="pricing-plan" id="business10">
<div class="badge-container">
<span class="badge">Recomended</span>
</div>
<h2>Business</h2>
<div class="price" id="business-price">
<strong>$49 /Month</strong><br>
Get 2 months free when billed annually!<br>
($59 /monthly)
</div>
<a href="https://YOUR_VCITA_DOMAIN/app/null?plan=business10&billing=monthly&current_plan=null">
<button class="upgrade-button">Get it now</button>
</a>
</div>
<div class="pricing-plan" id="business20">
<h2>Platinum</h2>
<div class="price" id="platinum-price">
<strong>$83 /Month</strong><br>
Get 2 months free when billed annually!<br>
($99 /monthly)
</div>
<a href="https://YOUR_VCITA_DOMAIN/app/null?plan=business20&billing=monthly&current_plan=null">
<button class="upgrade-button">Get it now</button>
</a>
</div>
</div>
<!-- Footer - Copyrights -->
<footer>
<span>©</span>
Copyright 2023 vcita Inc. All rights reserved.
</footer>
<script src="script.js"></script> <!-- Include your JavaScript file -->
</body></html>
Page JavaScript:
// Description: This file contains the logic for the pricing page.
// =====================================================================================================================
// Expected url params:
// su: The url to redirect to after a successful payment.
// cu: The url to redirect to after a canceled payment.
// plan: The current plan name. options are : essential, business (recommended), platinum.
// business_uid: The business uid. currently not in use!
// =====================================================================================================================
const urlParams = new URLSearchParams(window.location.search);
const baseUrl = "https://YOUR_VCITA_DOMAIN/app"
initPage();
function initPage(){
setPricesUrls();
setCancelUrl();
disableCurrentPlan();
}
function disableCurrentPlan(){
const currentPlanName = getUrlParamValue("plan")
const currentPlanContainer = document.getElementById(currentPlanName)
currentPlanContainer.classList.add("disabled")
// remove recommended badge
if (currentPlanName === "business") {
const badge = currentPlanContainer.getElementsByClassName("badge")[0]
badge.remove()
}
}
function setCancelUrl() {
const cancelePath = getUrlParamValue("cu")
const url = `${baseUrl}/${cancelePath}`
// get element
const headerContainers = document.getElementsByClassName("header")[0]
const linkElement = headerContainers.getElementsByTagName("a")[0]
linkElement.setAttribute("href", url)
}
function setPricesUrls() {
const planContainers = document.getElementsByClassName("pricing-plan")
for (let planContainer of planContainers) {
const selectedPlanName = planContainer.getAttribute("id")
const path = getUrlParamValue("su")
const url = buildPriceUrl(path, selectedPlanName)
const linkElement = planContainer.getElementsByTagName("a")[0]
linkElement.setAttribute("href", url)
}
return true
}
function buildPriceUrl(urlPath, plan) {
const currentPlanName = getUrlParamValue("plan")
url = `${baseUrl}/${urlPath}?plan=${plan}&billing=monthly¤t_plan=${currentPlanName}`;
return url;
}
function getUrlParamValue(paramName) {
return urlParams.get(paramName)
}
Access the complete example code on our Github repository here.
Updated about 1 month ago